Airbnb App Clone
React Native, Redux, Socket.IO
Airbnb App clone project. After completing the Airbnb site clone, I decided to clone the app using React Native. It was a solo project completed in less than three weeks.
What I Implemented
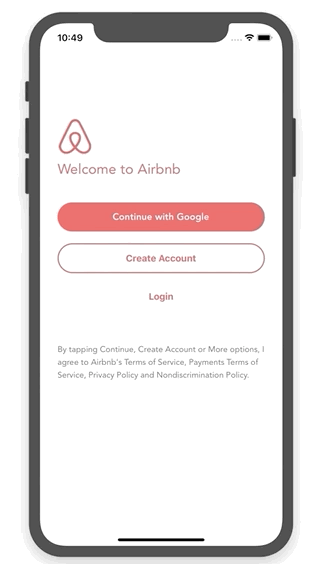
Signup and Login
- Created reusable input and form component
- Created reusable button component
- Implemented Yup and Joi for error handling
Homepage
- Created search filter
- Implemented Wix calendar picker library
- Created counter component
- Implemented redux to store user selected data
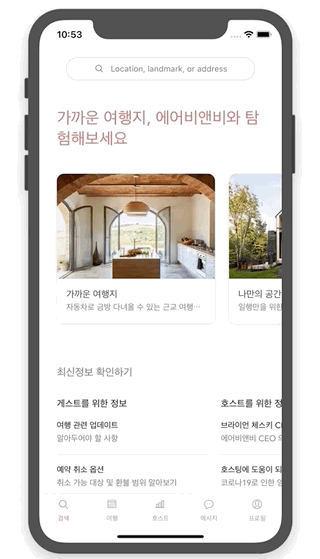
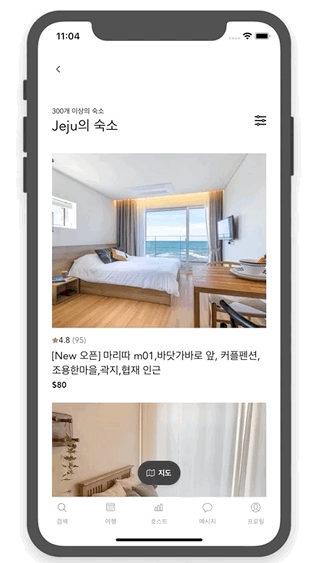
List and Details
- Created reusable card component
- Implemented Google maps
- Created custom image slider
Host
- Enabled adding image using native ImagePicker
- Enabled getting user's current location
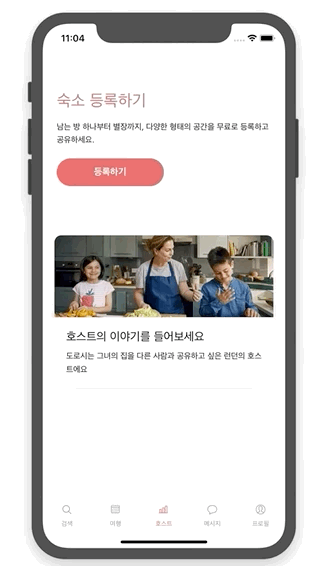
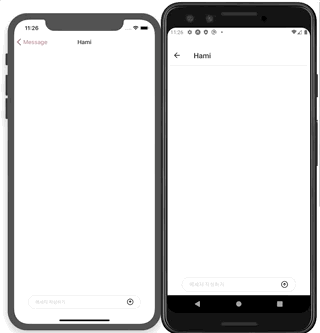
Messaging
- Creating socket connection
- Implemented live sending and receiving of messages
Features Componentized
Through this project, I learned the importance of reusable components. I tried to document them so that I can expand them into more projects later.
Buttons
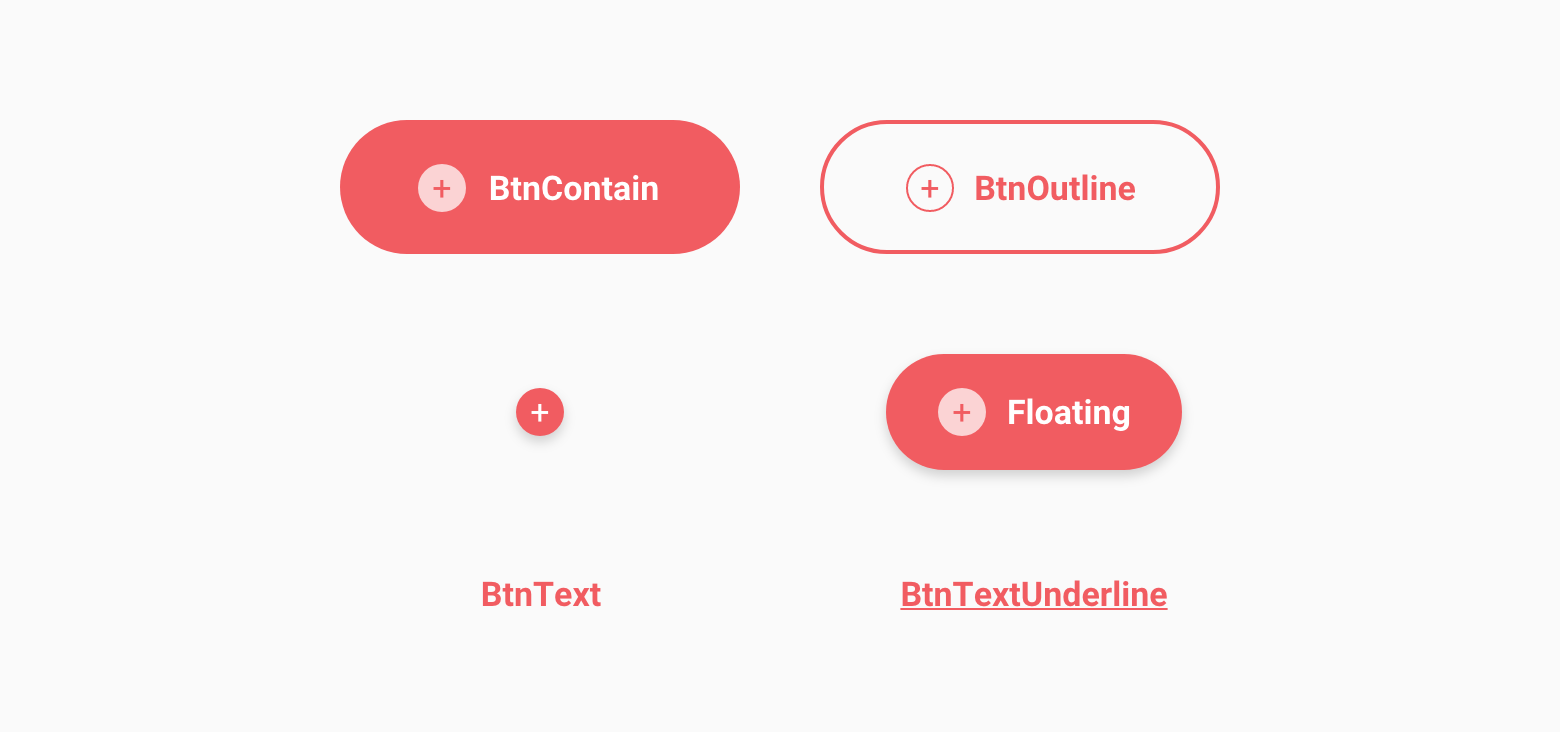
import * as Button from "../components/Button.js";
<Button.BtnContain
icon="search"
label="Next"
labelcolor={colors.white}
color={colors.red}
size="small"
disabled={false}
onPress={() => onNavigate()}
/>
Lists
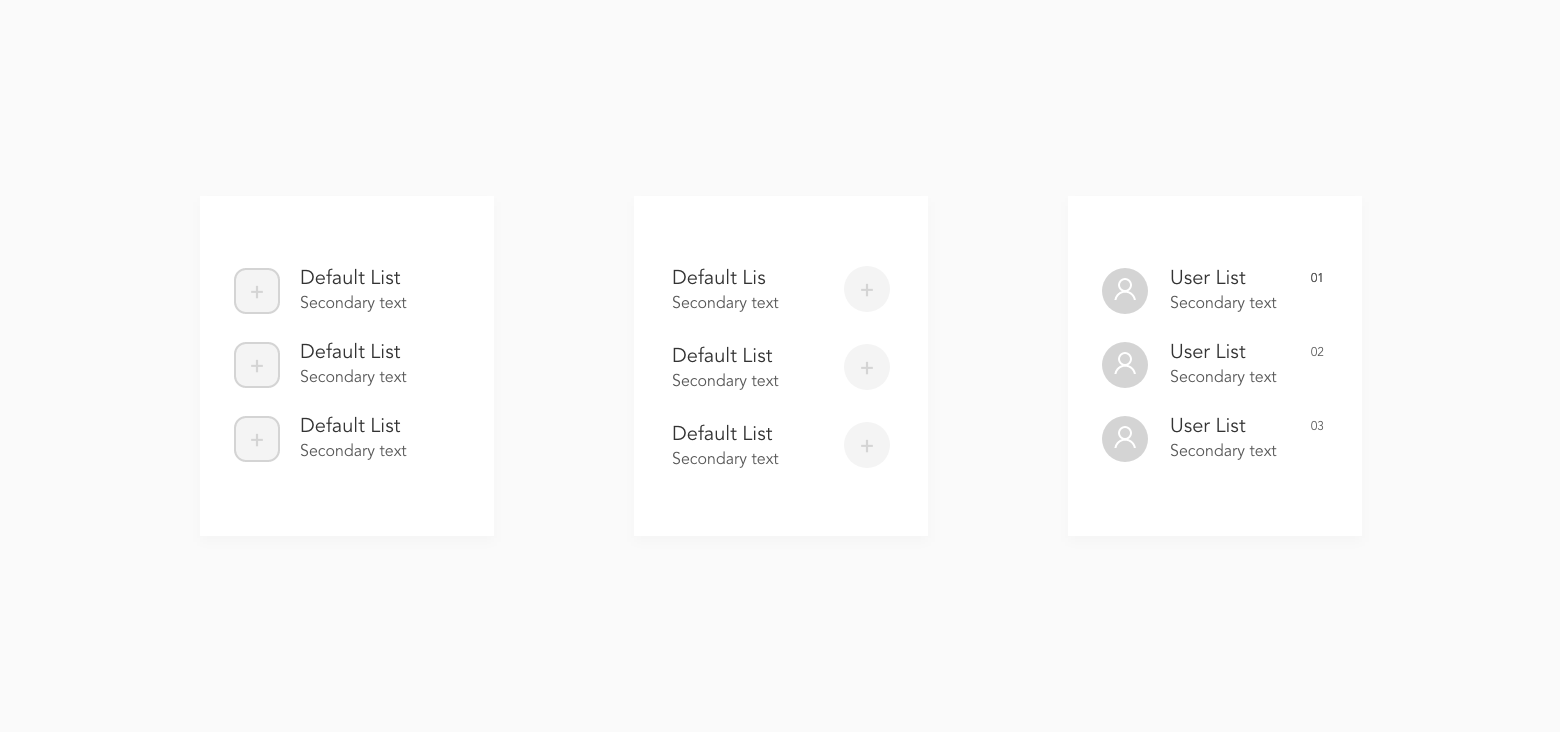
import * as List from "../components/List.js";
<ListItem.Default
title="Title"
secondary="Secondary Text"
containedicon="location"
onPress={() => onNavigate(item.title)}
/>
<List.UserList
title="User Name"
secondary="Secondary Text"
image={userimage}
meta={item.dates}
onPress={() => handleNavigation(item)}
RightActions={() => (
<DeleteItem onPress={() => handleDelete(item)} />
)}
/>
Cards
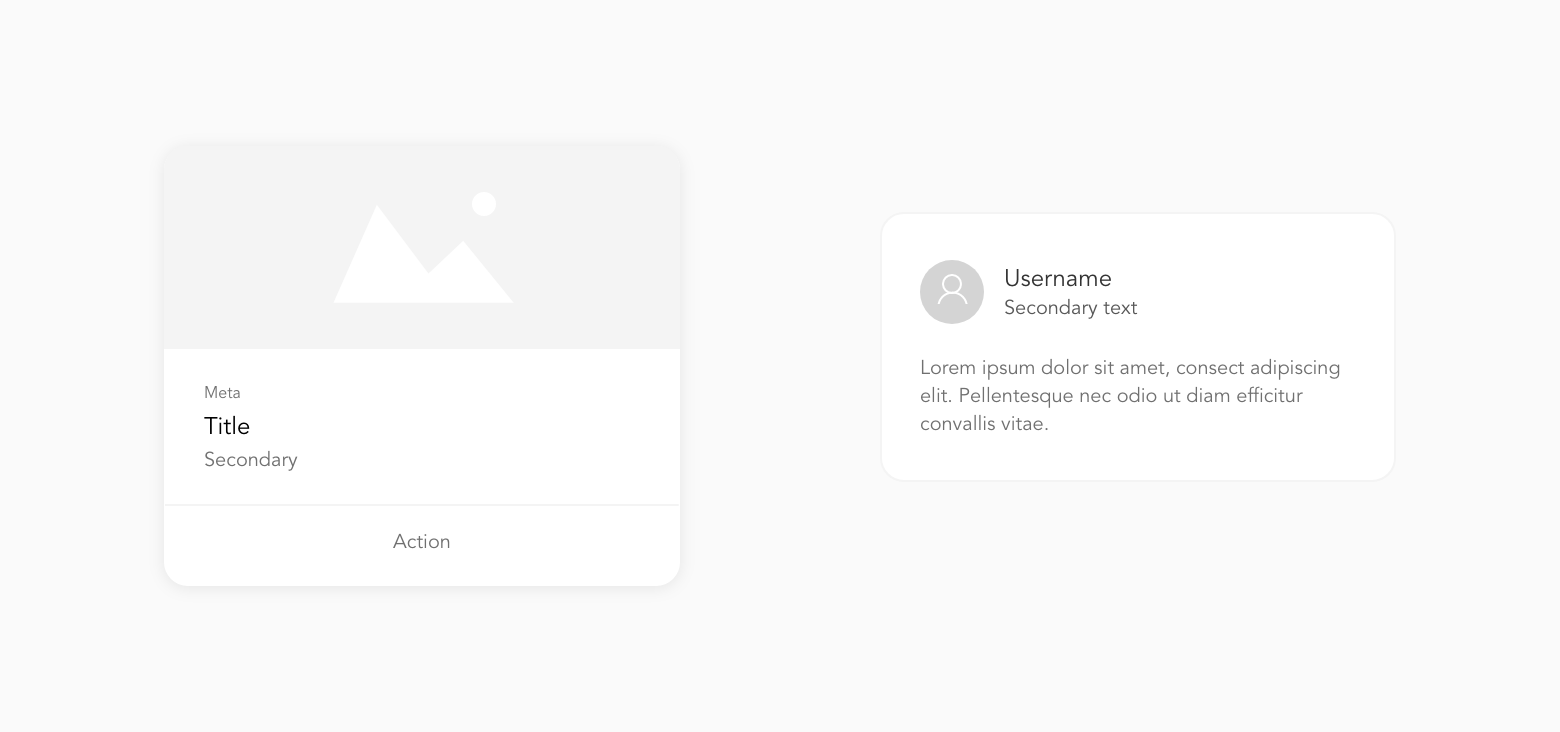
import * as Cards from "../components/Cards.js";
<Cards.Image
image={image}
sub="Subheader"
title="Title"
secondary="Secondary text"
action="View More"
onPress={() => console.log(item)}
/>